Pansharpening#
Landsat includes a panchromatic band which has greater spatial resolution (15 m versus the standard 30 m of visible bands). It can be used to artificially increase the resolution of the visible bands (red, green, and blue) in a process called pansharpening.
To illustrate this concept, let’s download a scene from just North of the city of Liverpool, UK:
import xlandsat as xls
import matplotlib.pyplot as plt
path = xls.datasets.fetch_liverpool()
path_pan = xls.datasets.fetch_liverpool_panchromatic()
Load the scene with xlandsat.load_scene
:
scene = xls.load_scene(path)
scene
<xarray.Dataset> Size: 2MB Dimensions: (easting: 433, northing: 267) Coordinates: * easting (easting) float64 3kB 4.87e+05 4.87e+05 ... 5e+05 5e+05 * northing (northing) float64 2kB 5.922e+06 5.922e+06 ... 5.93e+06 Data variables: coastal_aerosol (northing, easting) float16 231kB 0.06238 ... 0.0769 blue (northing, easting) float16 231kB 0.0708 ... 0.08301 green (northing, easting) float16 231kB 0.08618 ... 0.09753 red (northing, easting) float16 231kB 0.06824 0.06824 ... 0.116 nir (northing, easting) float16 231kB 0.04553 0.04553 ... 0.173 swir1 (northing, easting) float16 231kB 0.04626 ... 0.2213 swir2 (northing, easting) float16 231kB 0.047 0.04663 ... 0.1686 thermal (northing, easting) float16 231kB 287.0 287.0 ... 290.5 Attributes: (12/19) Conventions: CF-1.8 title: Landsat 8 scene from 2020-09-27 (path/row=204... digital_object_identifier: https://doi.org/10.5066/P9OGBGM6 origin: Image courtesy of the U.S. Geological Survey landsat_product_id: LC08_L2SP_204023_20200927_20201006_02_T1 processing_level: L2SP ... ... ellipsoid: WGS84 date_acquired: 2020-09-27 scene_center_time: 11:10:50.3140030Z wrs_path: 204 wrs_row: 23 mtl_file: GROUP = LANDSAT_METADATA_FILE\n GROUP = PROD...
xarray.Dataset
- easting: 433
- northing: 267
- easting(easting)float644.87e+05 4.87e+05 ... 5e+05 5e+05
- long_name :
- UTM easting
- standard_name :
- projection_x_coordinate
- units :
- m
array([487020., 487050., 487080., ..., 499920., 499950., 499980.])
- northing(northing)float645.922e+06 5.922e+06 ... 5.93e+06
- long_name :
- UTM northing
- standard_name :
- projection_y_coordinate
- units :
- m
array([5922000., 5922030., 5922060., ..., 5929920., 5929950., 5929980.])
- coastal_aerosol(northing, easting)float160.06238 0.06238 ... 0.07751 0.0769
- long_name :
- coastal aerosol
- units :
- reflectance
- number :
- 1
- filename :
- LC08_L2SP_204023_20200927_20201006_02_T1_SR_B1.TIF
- scaling_mult :
- 2e-05
- scaling_add :
- -0.1
array([[0.06238, 0.06238, 0.0625 , ..., 0.1581 , 0.1278 , 0.0862 ], [0.0631 , 0.06323, 0.06287, ..., 0.1318 , 0.10364, 0.07654], [0.06055, 0.0598 , 0.05957, ..., 0.135 , 0.0896 , 0.0774 ], ..., [0.0614 , 0.06165, 0.06128, ..., 0.07776, 0.0774 , 0.0773 ], [0.06104, 0.06104, 0.06165, ..., 0.0785 , 0.07654, 0.07837], [0.06238, 0.06116, 0.0625 , ..., 0.07996, 0.0775 , 0.0769 ]], dtype=float16)
- blue(northing, easting)float160.0708 0.07117 ... 0.08423 0.08301
- long_name :
- blue
- units :
- reflectance
- number :
- 2
- filename :
- LC08_L2SP_204023_20200927_20201006_02_T1_SR_B2.TIF
- scaling_mult :
- 2e-05
- scaling_add :
- -0.1
array([[0.0708 , 0.07117, 0.07117, ..., 0.1771 , 0.1381 , 0.0896 ], [0.0708 , 0.0709 , 0.0709 , ..., 0.1296 , 0.1068 , 0.07825], [0.06934, 0.0698 , 0.0697 , ..., 0.1554 , 0.0923 , 0.0774 ], ..., [0.0709 , 0.0708 , 0.07117, ..., 0.084 , 0.0835 , 0.08374], [0.0714 , 0.0707 , 0.0714 , ..., 0.0856 , 0.08215, 0.0834 ], [0.0714 , 0.0718 , 0.07166, ..., 0.0862 , 0.0842 , 0.083 ]], dtype=float16)
- green(northing, easting)float160.08618 0.08667 ... 0.09985 0.09753
- long_name :
- green
- units :
- reflectance
- number :
- 3
- filename :
- LC08_L2SP_204023_20200927_20201006_02_T1_SR_B3.TIF
- scaling_mult :
- 2e-05
- scaling_add :
- -0.1
array([[0.0862 , 0.0867 , 0.08655, ..., 0.2067 , 0.1556 , 0.10095], [0.0862 , 0.08655, 0.08655, ..., 0.137 , 0.11694, 0.09265], [0.0856 , 0.08545, 0.08545, ..., 0.1803 , 0.1046 , 0.08923], ..., [0.08826, 0.08777, 0.08826, ..., 0.1001 , 0.0985 , 0.09937], [0.0885 , 0.08826, 0.088 , ..., 0.10205, 0.0978 , 0.0978 ], [0.0885 , 0.0886 , 0.08813, ..., 0.1019 , 0.09985, 0.09753]], dtype=float16)
- red(northing, easting)float160.06824 0.06824 ... 0.1193 0.116
- long_name :
- red
- units :
- reflectance
- number :
- 4
- filename :
- LC08_L2SP_204023_20200927_20201006_02_T1_SR_B4.TIF
- scaling_mult :
- 2e-05
- scaling_add :
- -0.1
array([[0.06824, 0.06824, 0.0687 , ..., 0.2181 , 0.1649 , 0.10254], [0.06824, 0.06885, 0.06885, ..., 0.1451 , 0.11804, 0.0856 ], [0.06775, 0.06824, 0.0676 , ..., 0.1869 , 0.1113 , 0.0868 ], ..., [0.06934, 0.0692 , 0.0692 , ..., 0.12085, 0.1179 , 0.1185 ], [0.06934, 0.0691 , 0.0692 , ..., 0.12305, 0.11694, 0.1166 ], [0.0686 , 0.06885, 0.0687 , ..., 0.1185 , 0.11926, 0.11597]], dtype=float16)
- nir(northing, easting)float160.04553 0.04553 ... 0.1801 0.173
- long_name :
- near-infrared
- units :
- reflectance
- number :
- 5
- filename :
- LC08_L2SP_204023_20200927_20201006_02_T1_SR_B5.TIF
- scaling_mult :
- 2e-05
- scaling_add :
- -0.1
array([[0.04553, 0.04553, 0.04578, ..., 0.2335 , 0.1722 , 0.1571 ], [0.0454 , 0.04553, 0.04565, ..., 0.1893 , 0.1534 , 0.2052 ], [0.04492, 0.04492, 0.04504, ..., 0.1864 , 0.1534 , 0.1996 ], ..., [0.04395, 0.04395, 0.04382, ..., 0.1815 , 0.1774 , 0.1791 ], [0.04395, 0.04395, 0.0437 , ..., 0.1871 , 0.1732 , 0.1725 ], [0.0442 , 0.04395, 0.0437 , ..., 0.1888 , 0.18 , 0.173 ]], dtype=float16)
- swir1(northing, easting)float160.04626 0.04651 ... 0.2301 0.2213
- long_name :
- short-wave infrared 1
- units :
- reflectance
- number :
- 6
- filename :
- LC08_L2SP_204023_20200927_20201006_02_T1_SR_B6.TIF
- scaling_mult :
- 2e-05
- scaling_add :
- -0.1
array([[0.04626, 0.0465 , 0.0465 , ..., 0.2588 , 0.1891 , 0.1367 ], [0.04602, 0.04614, 0.0464 , ..., 0.2247 , 0.1632 , 0.1493 ], [0.04626, 0.04614, 0.0465 , ..., 0.1766 , 0.1333 , 0.1406 ], ..., [0.0459 , 0.04614, 0.04614, ..., 0.2228 , 0.2201 , 0.2203 ], [0.04602, 0.04614, 0.04614, ..., 0.2303 , 0.2198 , 0.2189 ], [0.0459 , 0.04614, 0.04614, ..., 0.2372 , 0.2301 , 0.2213 ]], dtype=float16)
- swir2(northing, easting)float160.047 0.04663 ... 0.1744 0.1686
- long_name :
- short-wave infrared 2
- units :
- reflectance
- number :
- 7
- filename :
- LC08_L2SP_204023_20200927_20201006_02_T1_SR_B7.TIF
- scaling_mult :
- 2e-05
- scaling_add :
- -0.1
array([[0.047 , 0.04663, 0.0465 , ..., 0.215 , 0.1613 , 0.1189 ], [0.04663, 0.0464 , 0.04626, ..., 0.1783 , 0.1422 , 0.10986], [0.04663, 0.0465 , 0.0465 , ..., 0.1649 , 0.1183 , 0.1134 ], ..., [0.04626, 0.0465 , 0.0464 , ..., 0.1678 , 0.1661 , 0.1659 ], [0.0465 , 0.0465 , 0.0465 , ..., 0.173 , 0.1664 , 0.1678 ], [0.0465 , 0.0465 , 0.0465 , ..., 0.1798 , 0.1744 , 0.1686 ]], dtype=float16)
- thermal(northing, easting)float16287.0 287.0 287.0 ... 290.5 290.5
- long_name :
- thermal
- units :
- Kelvin
- number :
- 10
- filename :
- LC08_L2SP_204023_20200927_20201006_02_T1_ST_B10.TIF
- scaling_mult :
- 0.00341802
- scaling_add :
- 149.0
array([[287. , 287. , 287. , ..., 291.5, 291.5, 291.5], [287. , 287. , 287. , ..., 291.5, 291.5, 291.5], [287. , 287. , 287. , ..., 291.5, 291.5, 291.5], ..., [286.5, 286.5, 286.5, ..., 290. , 290.2, 290.2], [286.5, 286.5, 286.5, ..., 290.2, 290.5, 290.5], [286.5, 286.5, 286.5, ..., 290.2, 290.5, 290.5]], dtype=float16)
- eastingPandasIndex
PandasIndex(Index([487020.0, 487050.0, 487080.0, 487110.0, 487140.0, 487170.0, 487200.0, 487230.0, 487260.0, 487290.0, ... 499710.0, 499740.0, 499770.0, 499800.0, 499830.0, 499860.0, 499890.0, 499920.0, 499950.0, 499980.0], dtype='float64', name='easting', length=433))
- northingPandasIndex
PandasIndex(Index([5922000.0, 5922030.0, 5922060.0, 5922090.0, 5922120.0, 5922150.0, 5922180.0, 5922210.0, 5922240.0, 5922270.0, ... 5929710.0, 5929740.0, 5929770.0, 5929800.0, 5929830.0, 5929860.0, 5929890.0, 5929920.0, 5929950.0, 5929980.0], dtype='float64', name='northing', length=267))
- Conventions :
- CF-1.8
- title :
- Landsat 8 scene from 2020-09-27 (path/row=204/23)
- digital_object_identifier :
- https://doi.org/10.5066/P9OGBGM6
- origin :
- Image courtesy of the U.S. Geological Survey
- landsat_product_id :
- LC08_L2SP_204023_20200927_20201006_02_T1
- processing_level :
- L2SP
- collection_number :
- 02
- collection_category :
- T1
- spacecraft_id :
- LANDSAT_8
- sensor_id :
- OLI_TIRS
- map_projection :
- UTM
- utm_zone :
- 30
- datum :
- WGS84
- ellipsoid :
- WGS84
- date_acquired :
- 2020-09-27
- scene_center_time :
- 11:10:50.3140030Z
- wrs_path :
- 204
- wrs_row :
- 23
- mtl_file :
- GROUP = LANDSAT_METADATA_FILE GROUP = PRODUCT_CONTENTS ORIGIN = "Image courtesy of the U.S. Geological Survey" DIGITAL_OBJECT_IDENTIFIER = "https://doi.org/10.5066/P9OGBGM6" LANDSAT_PRODUCT_ID = "LC08_L2SP_204023_20200927_20201006_02_T1" PROCESSING_LEVEL = "L2SP" COLLECTION_NUMBER = 02 COLLECTION_CATEGORY = "T1" OUTPUT_FORMAT = "GEOTIFF" FILE_NAME_BAND_1 = "LC08_L2SP_204023_20200927_20201006_02_T1_SR_B1.TIF" FILE_NAME_BAND_2 = "LC08_L2SP_204023_20200927_20201006_02_T1_SR_B2.TIF" FILE_NAME_BAND_3 = "LC08_L2SP_204023_20200927_20201006_02_T1_SR_B3.TIF" FILE_NAME_BAND_4 = "LC08_L2SP_204023_20200927_20201006_02_T1_SR_B4.TIF" FILE_NAME_BAND_5 = "LC08_L2SP_204023_20200927_20201006_02_T1_SR_B5.TIF" FILE_NAME_BAND_6 = "LC08_L2SP_204023_20200927_20201006_02_T1_SR_B6.TIF" FILE_NAME_BAND_7 = "LC08_L2SP_204023_20200927_20201006_02_T1_SR_B7.TIF" FILE_NAME_BAND_ST_B10 = "LC08_L2SP_204023_20200927_20201006_02_T1_ST_B10.TIF" FILE_NAME_THERMAL_RADIANCE = "LC08_L2SP_204023_20200927_20201006_02_T1_ST_TRAD.TIF" FILE_NAME_UPWELL_RADIANCE = "LC08_L2SP_204023_20200927_20201006_02_T1_ST_URAD.TIF" FILE_NAME_DOWNWELL_RADIANCE = "LC08_L2SP_204023_20200927_20201006_02_T1_ST_DRAD.TIF" FILE_NAME_ATMOSPHERIC_TRANSMITTANCE = "LC08_L2SP_204023_20200927_20201006_02_T1_ST_ATRAN.TIF" FILE_NAME_EMISSIVITY = "LC08_L2SP_204023_20200927_20201006_02_T1_ST_EMIS.TIF" FILE_NAME_EMISSIVITY_STDEV = "LC08_L2SP_204023_20200927_20201006_02_T1_ST_EMSD.TIF" FILE_NAME_CLOUD_DISTANCE = "LC08_L2SP_204023_20200927_20201006_02_T1_ST_CDIST.TIF" FILE_NAME_QUALITY_L2_AEROSOL = "LC08_L2SP_204023_20200927_20201006_02_T1_SR_QA_AEROSOL.TIF" FILE_NAME_QUALITY_L2_SURFACE_TEMPERATURE = "LC08_L2SP_204023_20200927_20201006_02_T1_ST_QA.TIF" FILE_NAME_QUALITY_L1_PIXEL = "LC08_L2SP_204023_20200927_20201006_02_T1_QA_PIXEL.TIF" FILE_NAME_QUALITY_L1_RADIOMETRIC_SATURATION = "LC08_L2SP_204023_20200927_20201006_02_T1_QA_RADSAT.TIF" FILE_NAME_ANGLE_COEFFICIENT = "LC08_L2SP_204023_20200927_20201006_02_T1_ANG.txt" FILE_NAME_METADATA_ODL = "LC08_L2SP_204023_20200927_20201006_02_T1_MTL.txt" FILE_NAME_METADATA_XML = "LC08_L2SP_204023_20200927_20201006_02_T1_MTL.xml" DATA_TYPE_BAND_1 = "UINT16" DATA_TYPE_BAND_2 = "UINT16" DATA_TYPE_BAND_3 = "UINT16" DATA_TYPE_BAND_4 = "UINT16" DATA_TYPE_BAND_5 = "UINT16" DATA_TYPE_BAND_6 = "UINT16" DATA_TYPE_BAND_7 = "UINT16" DATA_TYPE_BAND_ST_B10 = "UINT16" DATA_TYPE_THERMAL_RADIANCE = "INT16" DATA_TYPE_UPWELL_RADIANCE = "INT16" DATA_TYPE_DOWNWELL_RADIANCE = "INT16" DATA_TYPE_ATMOSPHERIC_TRANSMITTANCE = "INT16" DATA_TYPE_EMISSIVITY = "INT16" DATA_TYPE_EMISSIVITY_STDEV = "INT16" DATA_TYPE_CLOUD_DISTANCE = "INT16" DATA_TYPE_QUALITY_L2_AEROSOL = "UINT8" DATA_TYPE_QUALITY_L2_SURFACE_TEMPERATURE = "INT16" DATA_TYPE_QUALITY_L1_PIXEL = "UINT16" DATA_TYPE_QUALITY_L1_RADIOMETRIC_SATURATION = "UINT16" END_GROUP = PRODUCT_CONTENTS GROUP = IMAGE_ATTRIBUTES SPACECRAFT_ID = "LANDSAT_8" SENSOR_ID = "OLI_TIRS" WRS_TYPE = 2 WRS_PATH = 204 WRS_ROW = 23 NADIR_OFFNADIR = "NADIR" TARGET_WRS_PATH = 204 TARGET_WRS_ROW = 23 DATE_ACQUIRED = 2020-09-27 SCENE_CENTER_TIME = "11:10:50.3140030Z" STATION_ID = "LGN" CLOUD_COVER = 6.23 CLOUD_COVER_LAND = 1.37 IMAGE_QUALITY_OLI = 9 IMAGE_QUALITY_TIRS = 9 SATURATION_BAND_1 = "Y" SATURATION_BAND_2 = "Y" SATURATION_BAND_3 = "Y" SATURATION_BAND_4 = "Y" SATURATION_BAND_5 = "Y" SATURATION_BAND_6 = "Y" SATURATION_BAND_7 = "Y" SATURATION_BAND_8 = "N" SATURATION_BAND_9 = "N" ROLL_ANGLE = -0.001 SUN_AZIMUTH = 163.67259834 SUN_ELEVATION = 33.83332706 EARTH_SUN_DISTANCE = 1.0021760 TRUNCATION_OLI = "UPPER" TIRS_SSM_MODEL = "FINAL" TIRS_SSM_POSITION_STATUS = "ESTIMATED" END_GROUP = IMAGE_ATTRIBUTES GROUP = PROJECTION_ATTRIBUTES MAP_PROJECTION = "UTM" DATUM = "WGS84" ELLIPSOID = "WGS84" UTM_ZONE = 30 GRID_CELL_SIZE_REFLECTIVE = 30.00 GRID_CELL_SIZE_THERMAL = 30.00 REFLECTIVE_LINES = 267 REFLECTIVE_SAMPLES = 433 THERMAL_LINES = 267 THERMAL_SAMPLES = 433 ORIENTATION = "NORTH_UP" CORNER_UL_LAT_PRODUCT = 54.16532 CORNER_UL_LON_PRODUCT = -5.36506 CORNER_UR_LAT_PRODUCT = 54.18146 CORNER_UR_LON_PRODUCT = -1.69297 CORNER_LL_LAT_PRODUCT = 51.98808 CORNER_LL_LON_PRODUCT = -5.24861 CORNER_LR_LAT_PRODUCT = 52.00299 CORNER_LR_LON_PRODUCT = -1.75737 CORNER_UL_PROJECTION_X_PRODUCT = 487020.0 CORNER_UL_PROJECTION_Y_PRODUCT = 5929980.0 CORNER_UR_PROJECTION_X_PRODUCT = 499980.0 CORNER_UR_PROJECTION_Y_PRODUCT = 5929980.0 CORNER_LL_PROJECTION_X_PRODUCT = 487020.0 CORNER_LL_PROJECTION_Y_PRODUCT = 5922000.0 CORNER_LR_PROJECTION_X_PRODUCT = 499980.0 CORNER_LR_PROJECTION_Y_PRODUCT = 5922000.0 END_GROUP = PROJECTION_ATTRIBUTES GROUP = LEVEL2_PROCESSING_RECORD ORIGIN = "Image courtesy of the U.S. Geological Survey" DIGITAL_OBJECT_IDENTIFIER = "https://doi.org/10.5066/P9OGBGM6" REQUEST_ID = "P700g0pu7vqfu_00006" LANDSAT_PRODUCT_ID = "LC08_L2SP_204023_20200927_20201006_02_T1" PROCESSING_LEVEL = "L2SP" OUTPUT_FORMAT = "GEOTIFF" DATE_PRODUCT_GENERATED = 2020-10-06T10:42:07Z PROCESSING_SOFTWARE_VERSION = "LPGS_15.3.1" ALGORITHM_SOURCE_SURFACE_REFLECTANCE = "LaSRC_1.5.0" DATA_SOURCE_OZONE = "MODIS" DATA_SOURCE_PRESSURE = "Calculated" DATA_SOURCE_WATER_VAPOR = "MODIS" DATA_SOURCE_AIR_TEMPERATURE = "MODIS" ALGORITHM_SOURCE_SURFACE_TEMPERATURE = "st_1.3.0" DATA_SOURCE_REANALYSIS = "GEOS-5 FP-IT" END_GROUP = LEVEL2_PROCESSING_RECORD GROUP = LEVEL2_SURFACE_REFLECTANCE_PARAMETERS REFLECTANCE_MAXIMUM_BAND_1 = 1.602213 REFLECTANCE_MINIMUM_BAND_1 = -0.199972 REFLECTANCE_MAXIMUM_BAND_2 = 1.602213 REFLECTANCE_MINIMUM_BAND_2 = -0.199972 REFLECTANCE_MAXIMUM_BAND_3 = 1.602213 REFLECTANCE_MINIMUM_BAND_3 = -0.199972 REFLECTANCE_MAXIMUM_BAND_4 = 1.602213 REFLECTANCE_MINIMUM_BAND_4 = -0.199972 REFLECTANCE_MAXIMUM_BAND_5 = 1.602213 REFLECTANCE_MINIMUM_BAND_5 = -0.199972 REFLECTANCE_MAXIMUM_BAND_6 = 1.602213 REFLECTANCE_MINIMUM_BAND_6 = -0.199972 REFLECTANCE_MAXIMUM_BAND_7 = 1.602213 REFLECTANCE_MINIMUM_BAND_7 = -0.199972 QUANTIZE_CAL_MAX_BAND_1 = 65535 QUANTIZE_CAL_MIN_BAND_1 = 1 QUANTIZE_CAL_MAX_BAND_2 = 65535 QUANTIZE_CAL_MIN_BAND_2 = 1 QUANTIZE_CAL_MAX_BAND_3 = 65535 QUANTIZE_CAL_MIN_BAND_3 = 1 QUANTIZE_CAL_MAX_BAND_4 = 65535 QUANTIZE_CAL_MIN_BAND_4 = 1 QUANTIZE_CAL_MAX_BAND_5 = 65535 QUANTIZE_CAL_MIN_BAND_5 = 1 QUANTIZE_CAL_MAX_BAND_6 = 65535 QUANTIZE_CAL_MIN_BAND_6 = 1 QUANTIZE_CAL_MAX_BAND_7 = 65535 QUANTIZE_CAL_MIN_BAND_7 = 1 REFLECTANCE_MULT_BAND_1 = 2.75e-05 REFLECTANCE_MULT_BAND_2 = 2.75e-05 REFLECTANCE_MULT_BAND_3 = 2.75e-05 REFLECTANCE_MULT_BAND_4 = 2.75e-05 REFLECTANCE_MULT_BAND_5 = 2.75e-05 REFLECTANCE_MULT_BAND_6 = 2.75e-05 REFLECTANCE_MULT_BAND_7 = 2.75e-05 REFLECTANCE_ADD_BAND_1 = -0.2 REFLECTANCE_ADD_BAND_2 = -0.2 REFLECTANCE_ADD_BAND_3 = -0.2 REFLECTANCE_ADD_BAND_4 = -0.2 REFLECTANCE_ADD_BAND_5 = -0.2 REFLECTANCE_ADD_BAND_6 = -0.2 REFLECTANCE_ADD_BAND_7 = -0.2 END_GROUP = LEVEL2_SURFACE_REFLECTANCE_PARAMETERS GROUP = LEVEL2_SURFACE_TEMPERATURE_PARAMETERS TEMPERATURE_MAXIMUM_BAND_ST_B10 = 372.999941 TEMPERATURE_MINIMUM_BAND_ST_B10 = 149.003418 QUANTIZE_CAL_MAXIMUM_BAND_ST_B10 = 65535 QUANTIZE_CAL_MINIMUM_BAND_ST_B10 = 1 TEMPERATURE_MULT_BAND_ST_B10 = 0.00341802 TEMPERATURE_ADD_BAND_ST_B10 = 149.0 END_GROUP = LEVEL2_SURFACE_TEMPERATURE_PARAMETERS GROUP = LEVEL1_PROCESSING_RECORD ORIGIN = "Image courtesy of the U.S. Geological Survey" DIGITAL_OBJECT_IDENTIFIER = "https://doi.org/10.5066/P975CC9B" REQUEST_ID = "P700g0pu7vqfu_00006" LANDSAT_SCENE_ID = "LC82040232020271LGN00" LANDSAT_PRODUCT_ID = "LC08_L1TP_204023_20200927_20201006_02_T1" PROCESSING_LEVEL = "L1TP" COLLECTION_CATEGORY = "T1" OUTPUT_FORMAT = "GEOTIFF" DATE_PRODUCT_GENERATED = 2020-10-06T10:29:11Z PROCESSING_SOFTWARE_VERSION = "LPGS_15.3.1" FILE_NAME_BAND_1 = "LC08_L1TP_204023_20200927_20201006_02_T1_B1.TIF" FILE_NAME_BAND_2 = "LC08_L1TP_204023_20200927_20201006_02_T1_B2.TIF" FILE_NAME_BAND_3 = "LC08_L1TP_204023_20200927_20201006_02_T1_B3.TIF" FILE_NAME_BAND_4 = "LC08_L1TP_204023_20200927_20201006_02_T1_B4.TIF" FILE_NAME_BAND_5 = "LC08_L1TP_204023_20200927_20201006_02_T1_B5.TIF" FILE_NAME_BAND_6 = "LC08_L1TP_204023_20200927_20201006_02_T1_B6.TIF" FILE_NAME_BAND_7 = "LC08_L1TP_204023_20200927_20201006_02_T1_B7.TIF" FILE_NAME_BAND_8 = "LC08_L1TP_204023_20200927_20201006_02_T1_B8.TIF" FILE_NAME_BAND_9 = "LC08_L1TP_204023_20200927_20201006_02_T1_B9.TIF" FILE_NAME_BAND_10 = "LC08_L1TP_204023_20200927_20201006_02_T1_B10.TIF" FILE_NAME_BAND_11 = "LC08_L1TP_204023_20200927_20201006_02_T1_B11.TIF" FILE_NAME_QUALITY_L1_PIXEL = "LC08_L1TP_204023_20200927_20201006_02_T1_QA_PIXEL.TIF" FILE_NAME_QUALITY_L1_RADIOMETRIC_SATURATION = "LC08_L1TP_204023_20200927_20201006_02_T1_QA_RADSAT.TIF" FILE_NAME_ANGLE_COEFFICIENT = "LC08_L1TP_204023_20200927_20201006_02_T1_ANG.txt" FILE_NAME_ANGLE_SENSOR_AZIMUTH_BAND_4 = "LC08_L1TP_204023_20200927_20201006_02_T1_VAA.TIF" FILE_NAME_ANGLE_SENSOR_ZENITH_BAND_4 = "LC08_L1TP_204023_20200927_20201006_02_T1_VZA.TIF" FILE_NAME_ANGLE_SOLAR_AZIMUTH_BAND_4 = "LC08_L1TP_204023_20200927_20201006_02_T1_SAA.TIF" FILE_NAME_ANGLE_SOLAR_ZENITH_BAND_4 = "LC08_L1TP_204023_20200927_20201006_02_T1_SZA.TIF" FILE_NAME_METADATA_ODL = "LC08_L1TP_204023_20200927_20201006_02_T1_MTL.txt" FILE_NAME_METADATA_XML = "LC08_L1TP_204023_20200927_20201006_02_T1_MTL.xml" FILE_NAME_CPF = "LC08CPF_20200701_20200930_02.02" FILE_NAME_BPF_OLI = "LO8BPF20200927110102_20200927123956.01" FILE_NAME_BPF_TIRS = "LT8BPF20200916105511_20201002071648.01" FILE_NAME_RLUT = "LC08RLUT_20150303_20431231_02_01.h5" DATA_SOURCE_TIRS_STRAY_LIGHT_CORRECTION = "TIRS" DATA_SOURCE_ELEVATION = "GLS2000" GROUND_CONTROL_POINTS_VERSION = 5 GROUND_CONTROL_POINTS_MODEL = 840 GEOMETRIC_RMSE_MODEL = 6.318 GEOMETRIC_RMSE_MODEL_Y = 4.539 GEOMETRIC_RMSE_MODEL_X = 4.394 GROUND_CONTROL_POINTS_VERIFY = 171 GEOMETRIC_RMSE_VERIFY = 3.974 END_GROUP = LEVEL1_PROCESSING_RECORD GROUP = LEVEL1_MIN_MAX_RADIANCE RADIANCE_MAXIMUM_BAND_1 = 756.76562 RADIANCE_MINIMUM_BAND_1 = -62.49395 RADIANCE_MAXIMUM_BAND_2 = 774.93726 RADIANCE_MINIMUM_BAND_2 = -63.99457 RADIANCE_MAXIMUM_BAND_3 = 714.09802 RADIANCE_MINIMUM_BAND_3 = -58.97045 RADIANCE_MAXIMUM_BAND_4 = 602.16766 RADIANCE_MINIMUM_BAND_4 = -49.72720 RADIANCE_MAXIMUM_BAND_5 = 368.49658 RADIANCE_MINIMUM_BAND_5 = -30.43057 RADIANCE_MAXIMUM_BAND_6 = 91.64175 RADIANCE_MINIMUM_BAND_6 = -7.56781 RADIANCE_MAXIMUM_BAND_7 = 30.88818 RADIANCE_MINIMUM_BAND_7 = -2.55076 RADIANCE_MAXIMUM_BAND_8 = 681.48810 RADIANCE_MINIMUM_BAND_8 = -56.27751 RADIANCE_MAXIMUM_BAND_9 = 144.01683 RADIANCE_MINIMUM_BAND_9 = -11.89296 RADIANCE_MAXIMUM_BAND_10 = 22.00180 RADIANCE_MINIMUM_BAND_10 = 0.10033 RADIANCE_MAXIMUM_BAND_11 = 22.00180 RADIANCE_MINIMUM_BAND_11 = 0.10033 END_GROUP = LEVEL1_MIN_MAX_RADIANCE GROUP = LEVEL1_MIN_MAX_REFLECTANCE REFLECTANCE_MAXIMUM_BAND_1 = 1.210700 REFLECTANCE_MINIMUM_BAND_1 = -0.099980 REFLECTANCE_MAXIMUM_BAND_2 = 1.210700 REFLECTANCE_MINIMUM_BAND_2 = -0.099980 REFLECTANCE_MAXIMUM_BAND_3 = 1.210700 REFLECTANCE_MINIMUM_BAND_3 = -0.099980 REFLECTANCE_MAXIMUM_BAND_4 = 1.210700 REFLECTANCE_MINIMUM_BAND_4 = -0.099980 REFLECTANCE_MAXIMUM_BAND_5 = 1.210700 REFLECTANCE_MINIMUM_BAND_5 = -0.099980 REFLECTANCE_MAXIMUM_BAND_6 = 1.210700 REFLECTANCE_MINIMUM_BAND_6 = -0.099980 REFLECTANCE_MAXIMUM_BAND_7 = 1.210700 REFLECTANCE_MINIMUM_BAND_7 = -0.099980 REFLECTANCE_MAXIMUM_BAND_8 = 1.210700 REFLECTANCE_MINIMUM_BAND_8 = -0.099980 REFLECTANCE_MAXIMUM_BAND_9 = 1.210700 REFLECTANCE_MINIMUM_BAND_9 = -0.099980 END_GROUP = LEVEL1_MIN_MAX_REFLECTANCE GROUP = LEVEL1_MIN_MAX_PIXEL_VALUE QUANTIZE_CAL_MAX_BAND_1 = 65535 QUANTIZE_CAL_MIN_BAND_1 = 1 QUANTIZE_CAL_MAX_BAND_2 = 65535 QUANTIZE_CAL_MIN_BAND_2 = 1 QUANTIZE_CAL_MAX_BAND_3 = 65535 QUANTIZE_CAL_MIN_BAND_3 = 1 QUANTIZE_CAL_MAX_BAND_4 = 65535 QUANTIZE_CAL_MIN_BAND_4 = 1 QUANTIZE_CAL_MAX_BAND_5 = 65535 QUANTIZE_CAL_MIN_BAND_5 = 1 QUANTIZE_CAL_MAX_BAND_6 = 65535 QUANTIZE_CAL_MIN_BAND_6 = 1 QUANTIZE_CAL_MAX_BAND_7 = 65535 QUANTIZE_CAL_MIN_BAND_7 = 1 QUANTIZE_CAL_MAX_BAND_8 = 65535 QUANTIZE_CAL_MIN_BAND_8 = 1 QUANTIZE_CAL_MAX_BAND_9 = 65535 QUANTIZE_CAL_MIN_BAND_9 = 1 QUANTIZE_CAL_MAX_BAND_10 = 65535 QUANTIZE_CAL_MIN_BAND_10 = 1 QUANTIZE_CAL_MAX_BAND_11 = 65535 QUANTIZE_CAL_MIN_BAND_11 = 1 END_GROUP = LEVEL1_MIN_MAX_PIXEL_VALUE GROUP = LEVEL1_RADIOMETRIC_RESCALING RADIANCE_MULT_BAND_1 = 1.2501E-02 RADIANCE_MULT_BAND_2 = 1.2801E-02 RADIANCE_MULT_BAND_3 = 1.1796E-02 RADIANCE_MULT_BAND_4 = 9.9474E-03 RADIANCE_MULT_BAND_5 = 6.0873E-03 RADIANCE_MULT_BAND_6 = 1.5139E-03 RADIANCE_MULT_BAND_7 = 5.1025E-04 RADIANCE_MULT_BAND_8 = 1.1258E-02 RADIANCE_MULT_BAND_9 = 2.3791E-03 RADIANCE_MULT_BAND_10 = 3.3420E-04 RADIANCE_MULT_BAND_11 = 3.3420E-04 RADIANCE_ADD_BAND_1 = -62.50645 RADIANCE_ADD_BAND_2 = -64.00737 RADIANCE_ADD_BAND_3 = -58.98224 RADIANCE_ADD_BAND_4 = -49.73715 RADIANCE_ADD_BAND_5 = -30.43666 RADIANCE_ADD_BAND_6 = -7.56932 RADIANCE_ADD_BAND_7 = -2.55127 RADIANCE_ADD_BAND_8 = -56.28877 RADIANCE_ADD_BAND_9 = -11.89534 RADIANCE_ADD_BAND_10 = 0.10000 RADIANCE_ADD_BAND_11 = 0.10000 REFLECTANCE_MULT_BAND_1 = 2.0000E-05 REFLECTANCE_MULT_BAND_2 = 2.0000E-05 REFLECTANCE_MULT_BAND_3 = 2.0000E-05 REFLECTANCE_MULT_BAND_4 = 2.0000E-05 REFLECTANCE_MULT_BAND_5 = 2.0000E-05 REFLECTANCE_MULT_BAND_6 = 2.0000E-05 REFLECTANCE_MULT_BAND_7 = 2.0000E-05 REFLECTANCE_MULT_BAND_8 = 2.0000E-05 REFLECTANCE_MULT_BAND_9 = 2.0000E-05 REFLECTANCE_ADD_BAND_1 = -0.100000 REFLECTANCE_ADD_BAND_2 = -0.100000 REFLECTANCE_ADD_BAND_3 = -0.100000 REFLECTANCE_ADD_BAND_4 = -0.100000 REFLECTANCE_ADD_BAND_5 = -0.100000 REFLECTANCE_ADD_BAND_6 = -0.100000 REFLECTANCE_ADD_BAND_7 = -0.100000 REFLECTANCE_ADD_BAND_8 = -0.100000 REFLECTANCE_ADD_BAND_9 = -0.100000 END_GROUP = LEVEL1_RADIOMETRIC_RESCALING GROUP = LEVEL1_THERMAL_CONSTANTS K1_CONSTANT_BAND_10 = 774.8853 K2_CONSTANT_BAND_10 = 1321.0789 K1_CONSTANT_BAND_11 = 480.8883 K2_CONSTANT_BAND_11 = 1201.1442 END_GROUP = LEVEL1_THERMAL_CONSTANTS GROUP = LEVEL1_PROJECTION_PARAMETERS MAP_PROJECTION = "UTM" DATUM = "WGS84" ELLIPSOID = "WGS84" UTM_ZONE = 30 GRID_CELL_SIZE_PANCHROMATIC = 15.00 GRID_CELL_SIZE_REFLECTIVE = 30.00 GRID_CELL_SIZE_THERMAL = 30.00 ORIENTATION = "NORTH_UP" RESAMPLING_OPTION = "CUBIC_CONVOLUTION" END_GROUP = LEVEL1_PROJECTION_PARAMETERS END_GROUP = LANDSAT_METADATA_FILE END
And load the panchromatic band with xlandsat.load_panchromatic
:
panchromatic = xls.load_panchromatic(path_pan)
panchromatic
<xarray.DataArray 'pan' (northing: 534, easting: 867)> Size: 2MB array([[0.04228 , 0.04231999, 0.04229999, ..., 0.0603 , 0.0497 , 0.04848 ], [0.04224 , 0.04207999, 0.04212 , ..., 0.05284 , 0.04792 , 0.03968 ], [0.04251999, 0.04236 , 0.04207999, ..., 0.05194 , 0.04589999, 0.03664 ], ..., [0.04477999, 0.04421999, 0.04423999, ..., 0.05402 , 0.05444 , 0.05415999], [0.04465999, 0.04457999, 0.04387999, ..., 0.05435999, 0.05398 , 0.05471999], [0.04421999, 0.04408 , 0.04382 , ..., 0.05731999, 0.05505999, 0.05444 ]], dtype=float32) Coordinates: * easting (easting) float64 7kB 4.87e+05 4.87e+05 4.87e+05 ... 5e+05 5e+05 * northing (northing) float64 4kB 5.922e+06 5.922e+06 ... 5.93e+06 5.93e+06 Attributes: (12/25) long_name: panchromatic units: reflectance number: 8 filename: LC08_L1TP_204023_20200927_20201006_02_T1_B8.TIF scaling_mult: 2e-05 scaling_add: -0.1 ... ... ellipsoid: WGS84 date_acquired: 2020-09-27 scene_center_time: 11:10:50.3140030Z wrs_path: 204 wrs_row: 23 mtl_file: GROUP = LANDSAT_METADATA_FILE\n GROUP = PROD...
xarray.DataArray
'pan'
- northing: 534
- easting: 867
- 0.04228 0.04232 0.0423 0.0423 ... 0.05792 0.05732 0.05506 0.05444
array([[0.04228 , 0.04231999, 0.04229999, ..., 0.0603 , 0.0497 , 0.04848 ], [0.04224 , 0.04207999, 0.04212 , ..., 0.05284 , 0.04792 , 0.03968 ], [0.04251999, 0.04236 , 0.04207999, ..., 0.05194 , 0.04589999, 0.03664 ], ..., [0.04477999, 0.04421999, 0.04423999, ..., 0.05402 , 0.05444 , 0.05415999], [0.04465999, 0.04457999, 0.04387999, ..., 0.05435999, 0.05398 , 0.05471999], [0.04421999, 0.04408 , 0.04382 , ..., 0.05731999, 0.05505999, 0.05444 ]], dtype=float32)
- easting(easting)float644.87e+05 4.87e+05 ... 5e+05 5e+05
- long_name :
- UTM easting
- standard_name :
- projection_x_coordinate
- units :
- m
array([487005., 487020., 487035., ..., 499965., 499980., 499995.])
- northing(northing)float645.922e+06 5.922e+06 ... 5.93e+06
- long_name :
- UTM northing
- standard_name :
- projection_y_coordinate
- units :
- m
array([5922000., 5922015., 5922030., ..., 5929965., 5929980., 5929995.])
- eastingPandasIndex
PandasIndex(Index([487005.0, 487020.0, 487035.0, 487050.0, 487065.0, 487080.0, 487095.0, 487110.0, 487125.0, 487140.0, ... 499860.0, 499875.0, 499890.0, 499905.0, 499920.0, 499935.0, 499950.0, 499965.0, 499980.0, 499995.0], dtype='float64', name='easting', length=867))
- northingPandasIndex
PandasIndex(Index([5922000.0, 5922015.0, 5922030.0, 5922045.0, 5922060.0, 5922075.0, 5922090.0, 5922105.0, 5922120.0, 5922135.0, ... 5929860.0, 5929875.0, 5929890.0, 5929905.0, 5929920.0, 5929935.0, 5929950.0, 5929965.0, 5929980.0, 5929995.0], dtype='float64', name='northing', length=534))
- long_name :
- panchromatic
- units :
- reflectance
- number :
- 8
- filename :
- LC08_L1TP_204023_20200927_20201006_02_T1_B8.TIF
- scaling_mult :
- 2e-05
- scaling_add :
- -0.1
- Conventions :
- CF-1.8
- title :
- Landsat 8 panchromatic band from 2020-09-27 (path/row=204/23)
- digital_object_identifier :
- https://doi.org/10.5066/P975CC9B
- origin :
- Image courtesy of the U.S. Geological Survey
- landsat_product_id :
- LC08_L1TP_204023_20200927_20201006_02_T1
- processing_level :
- L1TP
- collection_number :
- 02
- collection_category :
- T1
- spacecraft_id :
- LANDSAT_8
- sensor_id :
- OLI_TIRS
- map_projection :
- UTM
- utm_zone :
- 30
- datum :
- WGS84
- ellipsoid :
- WGS84
- date_acquired :
- 2020-09-27
- scene_center_time :
- 11:10:50.3140030Z
- wrs_path :
- 204
- wrs_row :
- 23
- mtl_file :
- GROUP = LANDSAT_METADATA_FILE GROUP = PRODUCT_CONTENTS ORIGIN = "Image courtesy of the U.S. Geological Survey" DIGITAL_OBJECT_IDENTIFIER = "https://doi.org/10.5066/P975CC9B" LANDSAT_PRODUCT_ID = "LC08_L1TP_204023_20200927_20201006_02_T1" PROCESSING_LEVEL = "L1TP" COLLECTION_NUMBER = 02 COLLECTION_CATEGORY = "T1" OUTPUT_FORMAT = "GEOTIFF" FILE_NAME_BAND_1 = "LC08_L1TP_204023_20200927_20201006_02_T1_B1.TIF" FILE_NAME_BAND_2 = "LC08_L1TP_204023_20200927_20201006_02_T1_B2.TIF" FILE_NAME_BAND_3 = "LC08_L1TP_204023_20200927_20201006_02_T1_B3.TIF" FILE_NAME_BAND_4 = "LC08_L1TP_204023_20200927_20201006_02_T1_B4.TIF" FILE_NAME_BAND_5 = "LC08_L1TP_204023_20200927_20201006_02_T1_B5.TIF" FILE_NAME_BAND_6 = "LC08_L1TP_204023_20200927_20201006_02_T1_B6.TIF" FILE_NAME_BAND_7 = "LC08_L1TP_204023_20200927_20201006_02_T1_B7.TIF" FILE_NAME_BAND_8 = "LC08_L1TP_204023_20200927_20201006_02_T1_B8.TIF" FILE_NAME_BAND_9 = "LC08_L1TP_204023_20200927_20201006_02_T1_B9.TIF" FILE_NAME_BAND_10 = "LC08_L1TP_204023_20200927_20201006_02_T1_B10.TIF" FILE_NAME_BAND_11 = "LC08_L1TP_204023_20200927_20201006_02_T1_B11.TIF" FILE_NAME_QUALITY_L1_PIXEL = "LC08_L1TP_204023_20200927_20201006_02_T1_QA_PIXEL.TIF" FILE_NAME_QUALITY_L1_RADIOMETRIC_SATURATION = "LC08_L1TP_204023_20200927_20201006_02_T1_QA_RADSAT.TIF" FILE_NAME_ANGLE_COEFFICIENT = "LC08_L1TP_204023_20200927_20201006_02_T1_ANG.txt" FILE_NAME_ANGLE_SENSOR_AZIMUTH_BAND_4 = "LC08_L1TP_204023_20200927_20201006_02_T1_VAA.TIF" FILE_NAME_ANGLE_SENSOR_ZENITH_BAND_4 = "LC08_L1TP_204023_20200927_20201006_02_T1_VZA.TIF" FILE_NAME_ANGLE_SOLAR_AZIMUTH_BAND_4 = "LC08_L1TP_204023_20200927_20201006_02_T1_SAA.TIF" FILE_NAME_ANGLE_SOLAR_ZENITH_BAND_4 = "LC08_L1TP_204023_20200927_20201006_02_T1_SZA.TIF" FILE_NAME_METADATA_ODL = "LC08_L1TP_204023_20200927_20201006_02_T1_MTL.txt" FILE_NAME_METADATA_XML = "LC08_L1TP_204023_20200927_20201006_02_T1_MTL.xml" DATA_TYPE_BAND_1 = "UINT16" DATA_TYPE_BAND_2 = "UINT16" DATA_TYPE_BAND_3 = "UINT16" DATA_TYPE_BAND_4 = "UINT16" DATA_TYPE_BAND_5 = "UINT16" DATA_TYPE_BAND_6 = "UINT16" DATA_TYPE_BAND_7 = "UINT16" DATA_TYPE_BAND_8 = "UINT16" DATA_TYPE_BAND_9 = "UINT16" DATA_TYPE_BAND_10 = "UINT16" DATA_TYPE_BAND_11 = "UINT16" DATA_TYPE_QUALITY_L1_PIXEL = "UINT16" DATA_TYPE_QUALITY_L1_RADIOMETRIC_SATURATION = "UINT16" DATA_TYPE_ANGLE_SENSOR_AZIMUTH_BAND_4 = "INT16" DATA_TYPE_ANGLE_SENSOR_ZENITH_BAND_4 = "INT16" DATA_TYPE_ANGLE_SOLAR_AZIMUTH_BAND_4 = "INT16" DATA_TYPE_ANGLE_SOLAR_ZENITH_BAND_4 = "INT16" END_GROUP = PRODUCT_CONTENTS GROUP = IMAGE_ATTRIBUTES SPACECRAFT_ID = "LANDSAT_8" SENSOR_ID = "OLI_TIRS" WRS_TYPE = 2 WRS_PATH = 204 WRS_ROW = 23 NADIR_OFFNADIR = "NADIR" TARGET_WRS_PATH = 204 TARGET_WRS_ROW = 23 DATE_ACQUIRED = 2020-09-27 SCENE_CENTER_TIME = "11:10:50.3140030Z" STATION_ID = "LGN" CLOUD_COVER = 6.23 CLOUD_COVER_LAND = 1.37 IMAGE_QUALITY_OLI = 9 IMAGE_QUALITY_TIRS = 9 SATURATION_BAND_1 = "Y" SATURATION_BAND_2 = "Y" SATURATION_BAND_3 = "Y" SATURATION_BAND_4 = "Y" SATURATION_BAND_5 = "Y" SATURATION_BAND_6 = "Y" SATURATION_BAND_7 = "Y" SATURATION_BAND_8 = "N" SATURATION_BAND_9 = "N" ROLL_ANGLE = -0.001 SUN_AZIMUTH = 163.67259834 SUN_ELEVATION = 33.83332706 EARTH_SUN_DISTANCE = 1.0021760 TRUNCATION_OLI = "UPPER" TIRS_SSM_MODEL = "FINAL" TIRS_SSM_POSITION_STATUS = "ESTIMATED" END_GROUP = IMAGE_ATTRIBUTES GROUP = PROJECTION_ATTRIBUTES MAP_PROJECTION = "UTM" DATUM = "WGS84" ELLIPSOID = "WGS84" UTM_ZONE = 30 GRID_CELL_SIZE_PANCHROMATIC = 15.00 GRID_CELL_SIZE_REFLECTIVE = 30.00 GRID_CELL_SIZE_THERMAL = 30.00 PANCHROMATIC_LINES = 534 PANCHROMATIC_SAMPLES = 867 REFLECTIVE_LINES = 8081 REFLECTIVE_SAMPLES = 7991 THERMAL_LINES = 8081 THERMAL_SAMPLES = 7991 ORIENTATION = "NORTH_UP" CORNER_UL_LAT_PRODUCT = 54.16532 CORNER_UL_LON_PRODUCT = -5.36506 CORNER_UR_LAT_PRODUCT = 54.18146 CORNER_UR_LON_PRODUCT = -1.69297 CORNER_LL_LAT_PRODUCT = 51.98808 CORNER_LL_LON_PRODUCT = -5.24861 CORNER_LR_LAT_PRODUCT = 52.00299 CORNER_LR_LON_PRODUCT = -1.75737 CORNER_UL_PROJECTION_X_PRODUCT = 487005.0 CORNER_UL_PROJECTION_Y_PRODUCT = 5929995.0 CORNER_UR_PROJECTION_X_PRODUCT = 499995.0 CORNER_UR_PROJECTION_Y_PRODUCT = 5929995.0 CORNER_LL_PROJECTION_X_PRODUCT = 487005.0 CORNER_LL_PROJECTION_Y_PRODUCT = 5922000.0 CORNER_LR_PROJECTION_X_PRODUCT = 499995.0 CORNER_LR_PROJECTION_Y_PRODUCT = 5922000.0 END_GROUP = PROJECTION_ATTRIBUTES GROUP = LEVEL1_PROCESSING_RECORD ORIGIN = "Image courtesy of the U.S. Geological Survey" DIGITAL_OBJECT_IDENTIFIER = "https://doi.org/10.5066/P975CC9B" REQUEST_ID = "P700g0pu7vqfu_00006" LANDSAT_SCENE_ID = "LC82040232020271LGN00" LANDSAT_PRODUCT_ID = "LC08_L1TP_204023_20200927_20201006_02_T1" PROCESSING_LEVEL = "L1TP" COLLECTION_CATEGORY = "T1" OUTPUT_FORMAT = "GEOTIFF" DATE_PRODUCT_GENERATED = 2020-10-06T10:29:11Z PROCESSING_SOFTWARE_VERSION = "LPGS_15.3.1" FILE_NAME_BAND_1 = "LC08_L1TP_204023_20200927_20201006_02_T1_B1.TIF" FILE_NAME_BAND_2 = "LC08_L1TP_204023_20200927_20201006_02_T1_B2.TIF" FILE_NAME_BAND_3 = "LC08_L1TP_204023_20200927_20201006_02_T1_B3.TIF" FILE_NAME_BAND_4 = "LC08_L1TP_204023_20200927_20201006_02_T1_B4.TIF" FILE_NAME_BAND_5 = "LC08_L1TP_204023_20200927_20201006_02_T1_B5.TIF" FILE_NAME_BAND_6 = "LC08_L1TP_204023_20200927_20201006_02_T1_B6.TIF" FILE_NAME_BAND_7 = "LC08_L1TP_204023_20200927_20201006_02_T1_B7.TIF" FILE_NAME_BAND_8 = "LC08_L1TP_204023_20200927_20201006_02_T1_B8.TIF" FILE_NAME_BAND_9 = "LC08_L1TP_204023_20200927_20201006_02_T1_B9.TIF" FILE_NAME_BAND_10 = "LC08_L1TP_204023_20200927_20201006_02_T1_B10.TIF" FILE_NAME_BAND_11 = "LC08_L1TP_204023_20200927_20201006_02_T1_B11.TIF" FILE_NAME_QUALITY_L1_PIXEL = "LC08_L1TP_204023_20200927_20201006_02_T1_QA_PIXEL.TIF" FILE_NAME_QUALITY_L1_RADIOMETRIC_SATURATION = "LC08_L1TP_204023_20200927_20201006_02_T1_QA_RADSAT.TIF" FILE_NAME_ANGLE_COEFFICIENT = "LC08_L1TP_204023_20200927_20201006_02_T1_ANG.txt" FILE_NAME_ANGLE_SENSOR_AZIMUTH_BAND_4 = "LC08_L1TP_204023_20200927_20201006_02_T1_VAA.TIF" FILE_NAME_ANGLE_SENSOR_ZENITH_BAND_4 = "LC08_L1TP_204023_20200927_20201006_02_T1_VZA.TIF" FILE_NAME_ANGLE_SOLAR_AZIMUTH_BAND_4 = "LC08_L1TP_204023_20200927_20201006_02_T1_SAA.TIF" FILE_NAME_ANGLE_SOLAR_ZENITH_BAND_4 = "LC08_L1TP_204023_20200927_20201006_02_T1_SZA.TIF" FILE_NAME_METADATA_ODL = "LC08_L1TP_204023_20200927_20201006_02_T1_MTL.txt" FILE_NAME_METADATA_XML = "LC08_L1TP_204023_20200927_20201006_02_T1_MTL.xml" FILE_NAME_CPF = "LC08CPF_20200701_20200930_02.02" FILE_NAME_BPF_OLI = "LO8BPF20200927110102_20200927123956.01" FILE_NAME_BPF_TIRS = "LT8BPF20200916105511_20201002071648.01" FILE_NAME_RLUT = "LC08RLUT_20150303_20431231_02_01.h5" DATA_SOURCE_TIRS_STRAY_LIGHT_CORRECTION = "TIRS" DATA_SOURCE_ELEVATION = "GLS2000" GROUND_CONTROL_POINTS_VERSION = 5 GROUND_CONTROL_POINTS_MODEL = 840 GEOMETRIC_RMSE_MODEL = 6.318 GEOMETRIC_RMSE_MODEL_Y = 4.539 GEOMETRIC_RMSE_MODEL_X = 4.394 GROUND_CONTROL_POINTS_VERIFY = 171 GEOMETRIC_RMSE_VERIFY = 3.974 END_GROUP = LEVEL1_PROCESSING_RECORD GROUP = LEVEL1_MIN_MAX_RADIANCE RADIANCE_MAXIMUM_BAND_1 = 756.76562 RADIANCE_MINIMUM_BAND_1 = -62.49395 RADIANCE_MAXIMUM_BAND_2 = 774.93726 RADIANCE_MINIMUM_BAND_2 = -63.99457 RADIANCE_MAXIMUM_BAND_3 = 714.09802 RADIANCE_MINIMUM_BAND_3 = -58.97045 RADIANCE_MAXIMUM_BAND_4 = 602.16766 RADIANCE_MINIMUM_BAND_4 = -49.72720 RADIANCE_MAXIMUM_BAND_5 = 368.49658 RADIANCE_MINIMUM_BAND_5 = -30.43057 RADIANCE_MAXIMUM_BAND_6 = 91.64175 RADIANCE_MINIMUM_BAND_6 = -7.56781 RADIANCE_MAXIMUM_BAND_7 = 30.88818 RADIANCE_MINIMUM_BAND_7 = -2.55076 RADIANCE_MAXIMUM_BAND_8 = 681.48810 RADIANCE_MINIMUM_BAND_8 = -56.27751 RADIANCE_MAXIMUM_BAND_9 = 144.01683 RADIANCE_MINIMUM_BAND_9 = -11.89296 RADIANCE_MAXIMUM_BAND_10 = 22.00180 RADIANCE_MINIMUM_BAND_10 = 0.10033 RADIANCE_MAXIMUM_BAND_11 = 22.00180 RADIANCE_MINIMUM_BAND_11 = 0.10033 END_GROUP = LEVEL1_MIN_MAX_RADIANCE GROUP = LEVEL1_MIN_MAX_REFLECTANCE REFLECTANCE_MAXIMUM_BAND_1 = 1.210700 REFLECTANCE_MINIMUM_BAND_1 = -0.099980 REFLECTANCE_MAXIMUM_BAND_2 = 1.210700 REFLECTANCE_MINIMUM_BAND_2 = -0.099980 REFLECTANCE_MAXIMUM_BAND_3 = 1.210700 REFLECTANCE_MINIMUM_BAND_3 = -0.099980 REFLECTANCE_MAXIMUM_BAND_4 = 1.210700 REFLECTANCE_MINIMUM_BAND_4 = -0.099980 REFLECTANCE_MAXIMUM_BAND_5 = 1.210700 REFLECTANCE_MINIMUM_BAND_5 = -0.099980 REFLECTANCE_MAXIMUM_BAND_6 = 1.210700 REFLECTANCE_MINIMUM_BAND_6 = -0.099980 REFLECTANCE_MAXIMUM_BAND_7 = 1.210700 REFLECTANCE_MINIMUM_BAND_7 = -0.099980 REFLECTANCE_MAXIMUM_BAND_8 = 1.210700 REFLECTANCE_MINIMUM_BAND_8 = -0.099980 REFLECTANCE_MAXIMUM_BAND_9 = 1.210700 REFLECTANCE_MINIMUM_BAND_9 = -0.099980 END_GROUP = LEVEL1_MIN_MAX_REFLECTANCE GROUP = LEVEL1_MIN_MAX_PIXEL_VALUE QUANTIZE_CAL_MAX_BAND_1 = 65535 QUANTIZE_CAL_MIN_BAND_1 = 1 QUANTIZE_CAL_MAX_BAND_2 = 65535 QUANTIZE_CAL_MIN_BAND_2 = 1 QUANTIZE_CAL_MAX_BAND_3 = 65535 QUANTIZE_CAL_MIN_BAND_3 = 1 QUANTIZE_CAL_MAX_BAND_4 = 65535 QUANTIZE_CAL_MIN_BAND_4 = 1 QUANTIZE_CAL_MAX_BAND_5 = 65535 QUANTIZE_CAL_MIN_BAND_5 = 1 QUANTIZE_CAL_MAX_BAND_6 = 65535 QUANTIZE_CAL_MIN_BAND_6 = 1 QUANTIZE_CAL_MAX_BAND_7 = 65535 QUANTIZE_CAL_MIN_BAND_7 = 1 QUANTIZE_CAL_MAX_BAND_8 = 65535 QUANTIZE_CAL_MIN_BAND_8 = 1 QUANTIZE_CAL_MAX_BAND_9 = 65535 QUANTIZE_CAL_MIN_BAND_9 = 1 QUANTIZE_CAL_MAX_BAND_10 = 65535 QUANTIZE_CAL_MIN_BAND_10 = 1 QUANTIZE_CAL_MAX_BAND_11 = 65535 QUANTIZE_CAL_MIN_BAND_11 = 1 END_GROUP = LEVEL1_MIN_MAX_PIXEL_VALUE GROUP = LEVEL1_RADIOMETRIC_RESCALING RADIANCE_MULT_BAND_1 = 1.2501E-02 RADIANCE_MULT_BAND_2 = 1.2801E-02 RADIANCE_MULT_BAND_3 = 1.1796E-02 RADIANCE_MULT_BAND_4 = 9.9474E-03 RADIANCE_MULT_BAND_5 = 6.0873E-03 RADIANCE_MULT_BAND_6 = 1.5139E-03 RADIANCE_MULT_BAND_7 = 5.1025E-04 RADIANCE_MULT_BAND_8 = 1.1258E-02 RADIANCE_MULT_BAND_9 = 2.3791E-03 RADIANCE_MULT_BAND_10 = 3.3420E-04 RADIANCE_MULT_BAND_11 = 3.3420E-04 RADIANCE_ADD_BAND_1 = -62.50645 RADIANCE_ADD_BAND_2 = -64.00737 RADIANCE_ADD_BAND_3 = -58.98224 RADIANCE_ADD_BAND_4 = -49.73715 RADIANCE_ADD_BAND_5 = -30.43666 RADIANCE_ADD_BAND_6 = -7.56932 RADIANCE_ADD_BAND_7 = -2.55127 RADIANCE_ADD_BAND_8 = -56.28877 RADIANCE_ADD_BAND_9 = -11.89534 RADIANCE_ADD_BAND_10 = 0.10000 RADIANCE_ADD_BAND_11 = 0.10000 REFLECTANCE_MULT_BAND_1 = 2.0000E-05 REFLECTANCE_MULT_BAND_2 = 2.0000E-05 REFLECTANCE_MULT_BAND_3 = 2.0000E-05 REFLECTANCE_MULT_BAND_4 = 2.0000E-05 REFLECTANCE_MULT_BAND_5 = 2.0000E-05 REFLECTANCE_MULT_BAND_6 = 2.0000E-05 REFLECTANCE_MULT_BAND_7 = 2.0000E-05 REFLECTANCE_MULT_BAND_8 = 2.0000E-05 REFLECTANCE_MULT_BAND_9 = 2.0000E-05 REFLECTANCE_ADD_BAND_1 = -0.100000 REFLECTANCE_ADD_BAND_2 = -0.100000 REFLECTANCE_ADD_BAND_3 = -0.100000 REFLECTANCE_ADD_BAND_4 = -0.100000 REFLECTANCE_ADD_BAND_5 = -0.100000 REFLECTANCE_ADD_BAND_6 = -0.100000 REFLECTANCE_ADD_BAND_7 = -0.100000 REFLECTANCE_ADD_BAND_8 = -0.100000 REFLECTANCE_ADD_BAND_9 = -0.100000 END_GROUP = LEVEL1_RADIOMETRIC_RESCALING GROUP = LEVEL1_THERMAL_CONSTANTS K1_CONSTANT_BAND_10 = 774.8853 K2_CONSTANT_BAND_10 = 1321.0789 K1_CONSTANT_BAND_11 = 480.8883 K2_CONSTANT_BAND_11 = 1201.1442 END_GROUP = LEVEL1_THERMAL_CONSTANTS GROUP = LEVEL1_PROJECTION_PARAMETERS MAP_PROJECTION = "UTM" DATUM = "WGS84" ELLIPSOID = "WGS84" UTM_ZONE = 30 GRID_CELL_SIZE_PANCHROMATIC = 15.00 GRID_CELL_SIZE_REFLECTIVE = 30.00 GRID_CELL_SIZE_THERMAL = 30.00 ORIENTATION = "NORTH_UP" RESAMPLING_OPTION = "CUBIC_CONVOLUTION" END_GROUP = LEVEL1_PROJECTION_PARAMETERS END_GROUP = LANDSAT_METADATA_FILE END
Now we can plot an RGB composite and the panchromatic band for comparison:
rgb = xls.composite(scene, rescale_to=(0, 0.25))
plt.figure(figsize=(16, 10))
ax = plt.subplot(2, 1, 1)
rgb.plot.imshow(ax=ax)
ax.set_aspect("equal")
ax.set_title("RGB")
ax = plt.subplot(2, 1, 2)
panchromatic.plot.pcolormesh(
ax=ax, cmap="gray", vmin=0.02, vmax=0.1, add_colorbar=False,
)
ax.set_aspect("equal")
ax.set_title("Panchromatic")
plt.tight_layout()
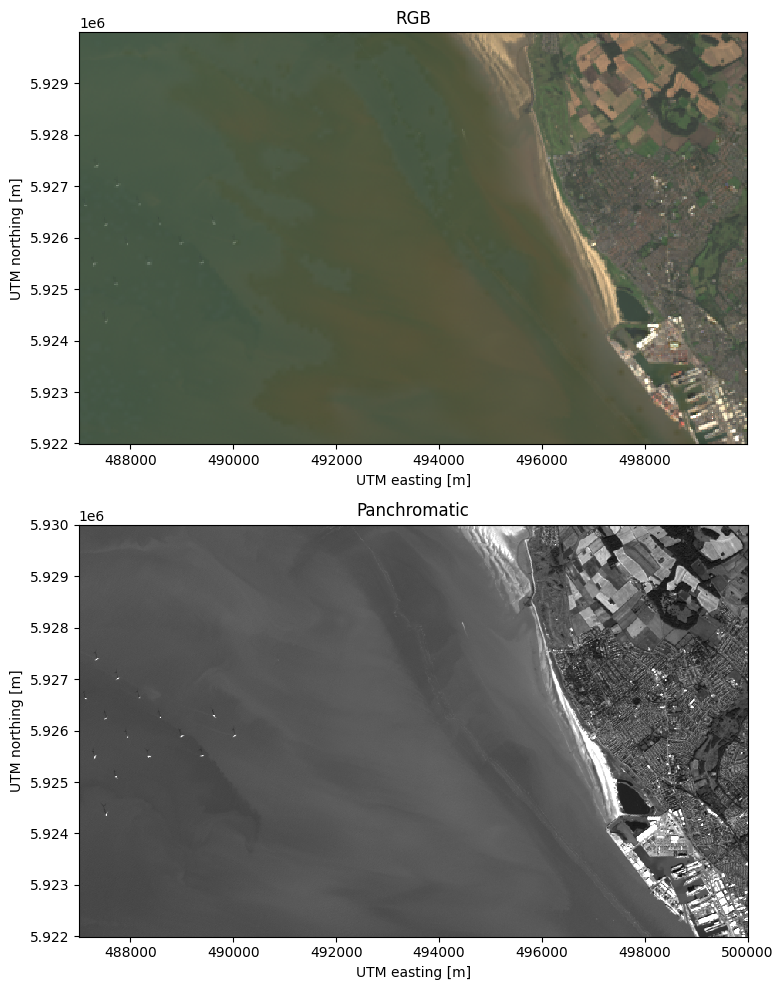
The pansharpening is implemented in xlandsat.pansharpen
:
scene_sharp = xls.pansharpen(scene, panchromatic)
scene_sharp
<xarray.Dataset> Size: 6MB Dimensions: (northing: 534, easting: 867) Coordinates: * northing (northing) float64 4kB 5.922e+06 5.922e+06 ... 5.93e+06 5.93e+06 * easting (easting) float64 7kB 4.87e+05 4.87e+05 4.87e+05 ... 5e+05 5e+05 Data variables: red (northing, easting) float32 2MB 0.03763 0.03767 ... 0.06035 green (northing, easting) float32 2MB 0.04753 0.04758 ... 0.05076 blue (northing, easting) float32 2MB 0.03905 0.03909 ... 0.04369 0.0432 Attributes: (12/21) Conventions: CF-1.8 title: Pansharpend Landsat 8 scene from 2020-09-27... digital_object_identifier: https://doi.org/10.5066/P9OGBGM6 origin: Image courtesy of the U.S. Geological Survey landsat_product_id: LC08_L2SP_204023_20200927_20201006_02_T1 processing_level: L2SP ... ... scene_center_time: 11:10:50.3140030Z wrs_path: 204 wrs_row: 23 pansharpening_method: Weighted Brovey Transform pansharpening_rgb_weights: (1, 1, 0.2) pansharpening_band_filename: LC08_L1TP_204023_20200927_20201006_02_T1_B8...
xarray.Dataset
- northing: 534
- easting: 867
- northing(northing)float645.922e+06 5.922e+06 ... 5.93e+06
- long_name :
- UTM northing
- standard_name :
- projection_y_coordinate
- units :
- m
array([5922000., 5922015., 5922030., ..., 5929965., 5929980., 5929995.])
- easting(easting)float644.87e+05 4.87e+05 ... 5e+05 5e+05
- long_name :
- UTM easting
- standard_name :
- projection_x_coordinate
- units :
- m
array([487005., 487020., 487035., ..., 499965., 499980., 499995.])
- red(northing, easting)float320.03763 0.03767 ... 0.06103 0.06035
- long_name :
- red
- units :
- reflectance
- number :
- 4
- filename :
- LC08_L2SP_204023_20200927_20201006_02_T1_SR_B4.TIF
- scaling_mult :
- 2e-05
- scaling_add :
- -0.1
array([[0.03763458, 0.03767018, 0.03765238, ..., 0.06281056, 0.05060364, 0.04936145], [0.03759898, 0.03745655, 0.03749217, ..., 0.05503997, 0.04879127, 0.04040145], [0.03784821, 0.03770579, 0.03745655, ..., 0.05259265, 0.04456495, 0.03557429], ..., [0.03968024, 0.03918402, 0.03920174, ..., 0.06010587, 0.06038351, 0.06007293], [0.03930919, 0.03923877, 0.03862264, ..., 0.06042061, 0.05983781, 0.0606581 ], [0.03892191, 0.03879868, 0.03856984, ..., 0.06371062, 0.061035 , 0.06034772]], dtype=float32)
- green(northing, easting)float320.04753 0.04758 ... 0.05133 0.05076
- long_name :
- green
- units :
- reflectance
- number :
- 3
- filename :
- LC08_L2SP_204023_20200927_20201006_02_T1_SR_B3.TIF
- scaling_mult :
- 2e-05
- scaling_add :
- -0.1
array([[0.04753133, 0.04757629, 0.04755381, ..., 0.05927718, 0.04982048, 0.04859752], [0.04748637, 0.04730649, 0.04735147, ..., 0.05194372, 0.04803617, 0.03977619], [0.04780114, 0.04762128, 0.04730649, ..., 0.05210317, 0.04825221, 0.03851767], ..., [0.0506482 , 0.05001481, 0.05003743, ..., 0.05025553, 0.05064627, 0.05038578], [0.05071025, 0.05061941, 0.04982458, ..., 0.05058757, 0.05032675, 0.05101666], [0.05021064, 0.05005168, 0.04975646, ..., 0.05334215, 0.05133365, 0.05075561]], dtype=float32)
- blue(northing, easting)float320.03905 0.03909 ... 0.04369 0.0432
- long_name :
- blue
- units :
- reflectance
- number :
- 2
- filename :
- LC08_L2SP_204023_20200927_20201006_02_T1_SR_B2.TIF
- scaling_mult :
- 2e-05
- scaling_add :
- -0.1
array([[0.0390484 , 0.03908534, 0.03906687, ..., 0.05258235, 0.04421794, 0.04313251], [0.03901147, 0.03886368, 0.03890064, ..., 0.04607714, 0.04263428, 0.03530318], [0.03927006, 0.03912229, 0.03886368, ..., 0.04758901, 0.04075055, 0.03252941], ..., [0.04086786, 0.04035678, 0.04037503, ..., 0.04222469, 0.04318527, 0.04296315], [0.04091793, 0.04084463, 0.04020328, ..., 0.04267167, 0.04283128, 0.04341843], [0.04051479, 0.04038653, 0.04014832, ..., 0.04499521, 0.04368821, 0.04319626]], dtype=float32)
- northingPandasIndex
PandasIndex(Index([5922000.0, 5922015.0, 5922030.0, 5922045.0, 5922060.0, 5922075.0, 5922090.0, 5922105.0, 5922120.0, 5922135.0, ... 5929860.0, 5929875.0, 5929890.0, 5929905.0, 5929920.0, 5929935.0, 5929950.0, 5929965.0, 5929980.0, 5929995.0], dtype='float64', name='northing', length=534))
- eastingPandasIndex
PandasIndex(Index([487005.0, 487020.0, 487035.0, 487050.0, 487065.0, 487080.0, 487095.0, 487110.0, 487125.0, 487140.0, ... 499860.0, 499875.0, 499890.0, 499905.0, 499920.0, 499935.0, 499950.0, 499965.0, 499980.0, 499995.0], dtype='float64', name='easting', length=867))
- Conventions :
- CF-1.8
- title :
- Pansharpend Landsat 8 scene from 2020-09-27 (path/row=204/23)
- digital_object_identifier :
- https://doi.org/10.5066/P9OGBGM6
- origin :
- Image courtesy of the U.S. Geological Survey
- landsat_product_id :
- LC08_L2SP_204023_20200927_20201006_02_T1
- processing_level :
- L2SP
- collection_number :
- 02
- collection_category :
- T1
- spacecraft_id :
- LANDSAT_8
- sensor_id :
- OLI_TIRS
- map_projection :
- UTM
- utm_zone :
- 30
- datum :
- WGS84
- ellipsoid :
- WGS84
- date_acquired :
- 2020-09-27
- scene_center_time :
- 11:10:50.3140030Z
- wrs_path :
- 204
- wrs_row :
- 23
- pansharpening_method :
- Weighted Brovey Transform
- pansharpening_rgb_weights :
- (1, 1, 0.2)
- pansharpening_band_filename :
- LC08_L1TP_204023_20200927_20201006_02_T1_B8.TIF
Finally, let’s compare the sharpened and original RGB composites:
rgb_sharp = xls.composite(scene_sharp, rescale_to=(0, 0.15))
plt.figure(figsize=(16, 10))
ax = plt.subplot(2, 1, 1)
rgb.plot.imshow(ax=ax)
ax.set_aspect("equal")
ax.set_title("Original")
ax = plt.subplot(2, 1, 2)
rgb_sharp.plot.imshow(ax=ax)
ax.set_aspect("equal")
ax.set_title("Pansharpened")
plt.tight_layout()
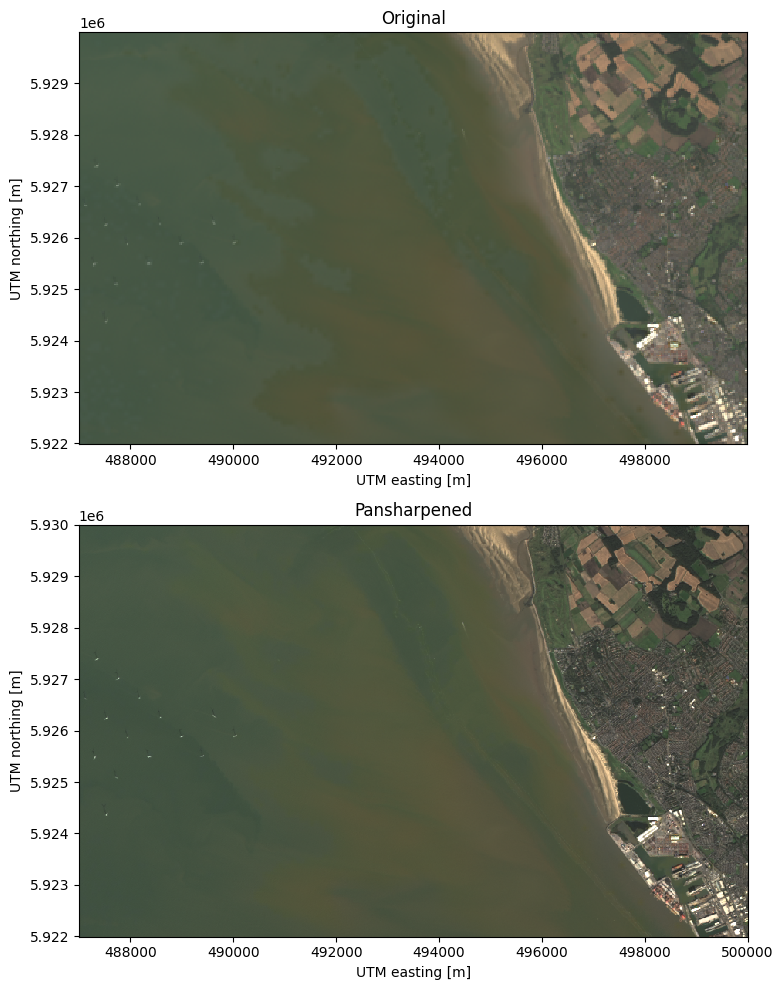